반응형
# SpringBoot에 스케줄을 적용해서 정기적으로 데이터를 CRUD 해보자.
- 일단 스프링은 기능이 참 많은거 같다... 어노테이션...
시작해보자.
1. 일단 main 클래스에 스케줄 어노테이션을 붙이자.
- 복수의 클래스에 아래의 어노테이션이 있어도 무관하더라.
@EnableScheduling
@EnableBatchProcessing // 배치 기능 활성화
2. Schedule 컨트롤러를 만들자.
@Slf4j
@RestController
public class ScheduleController {
@Autowired
private ScheduleMapper scheduleMapper;
@Scheduled(cron = "* * * * * *", zone = "Asia/Seoul")
@PostMapping("/insert")
public void scheduledInsert() throws Exception {
scheduleMapper.insertValue();
log.info("배치 입니다.");
}
3. DTO를 만들자.
public class ScheduleDto {
public String name;
public String location;
public String initial;
public void setName() {
this.name = name;
}
public String getName() {
return name;
}
public void setLocation() {
this.location = location;
}
public String getLocation() {
return location;
}
public void setInitial() {
this.initial = initial;
}
public String getInitial() {
return initial;
}
}
- Lombok 버전
@Getter
@Setter
public class ScheduleDto {
private String name;
private String location;
private String initial;
}
4. Mapper를 만들자.
- 중간에 service를 거쳐서 Mapper로 가는게 낫긴 하지만 service 없이 바로 mapper에서 데이터를 가져오자.
@Repository
public interface ScheduleMapper {
List<ScheduleDto> getScheduleValue() throws Exception;
int insertValue () throws Exception;
}
5. xml 파일을 생성, mybatis 쿼리를 넣자.
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.test.mapper.ScheduleMapper">
<select id="getScheduleValue" resultType="com.test.dto.ScheduleDto" fetchSize="250">
SELECT name, location, initial
FROM public.base_data
</select>
<insert id="insertValue" >
INSERT INTO public.base_data(
name, location, initial)
VALUES ('김승현', '서울', '짱구');
</insert>
</mapper>
6. 프로그램을 실행하고 DB 테이블을 확인해보자.
- 1초에 한번씩 해당 메소드를 실행하도록 설정 했으므로 아래와 같이 1초에 한번씩 데이터가 Insert 되는것을 볼 수 있다.
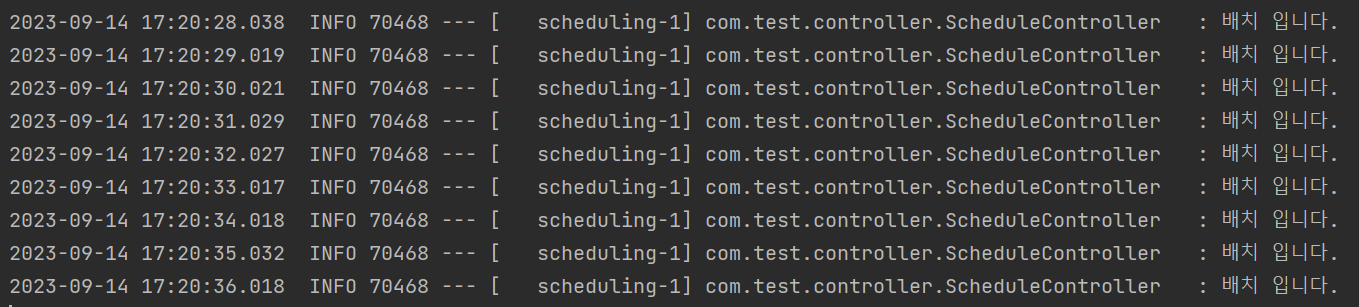
- Postman에서 데이터를 조회해보자.
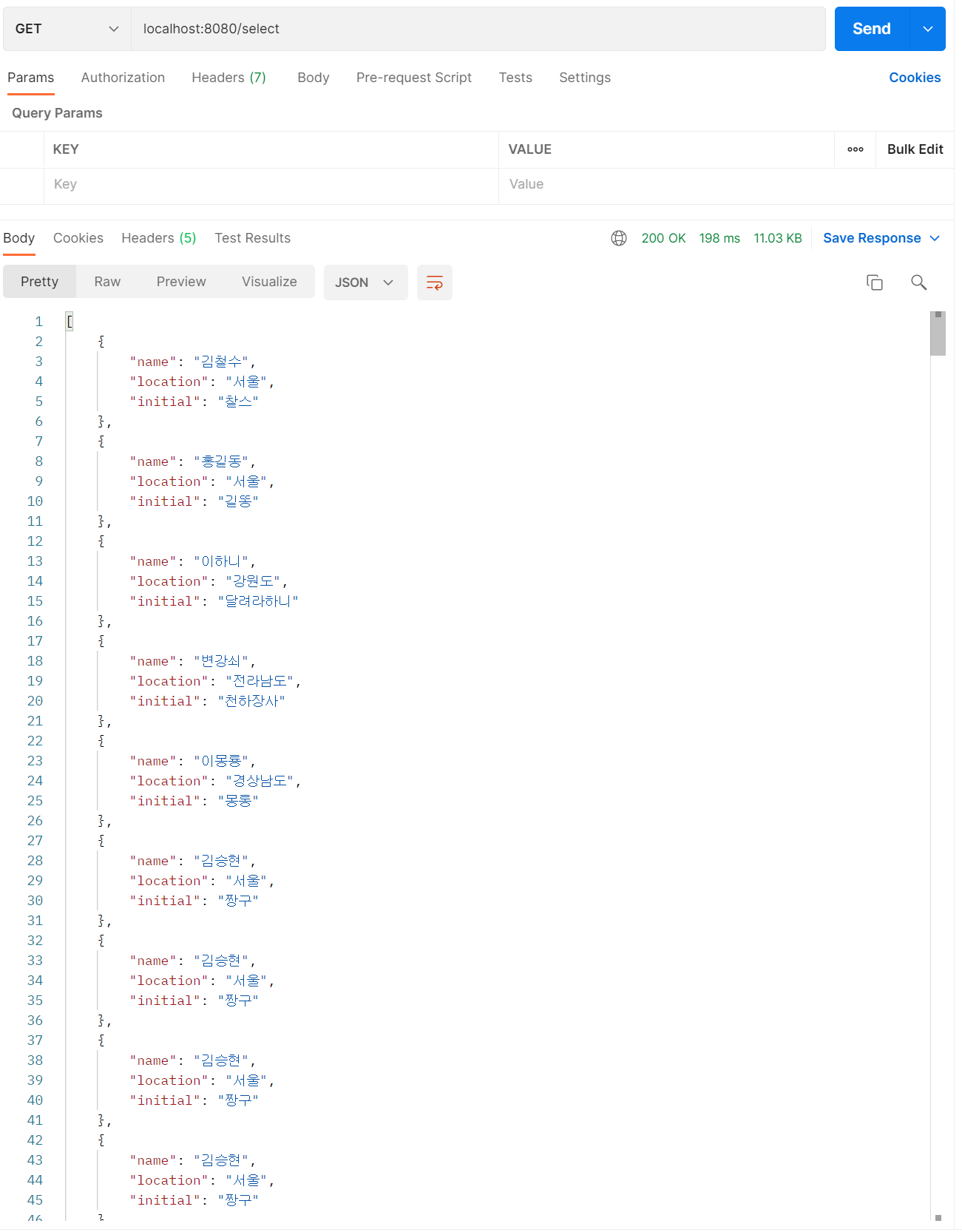
7. 실행 화면은 아래와 같다.
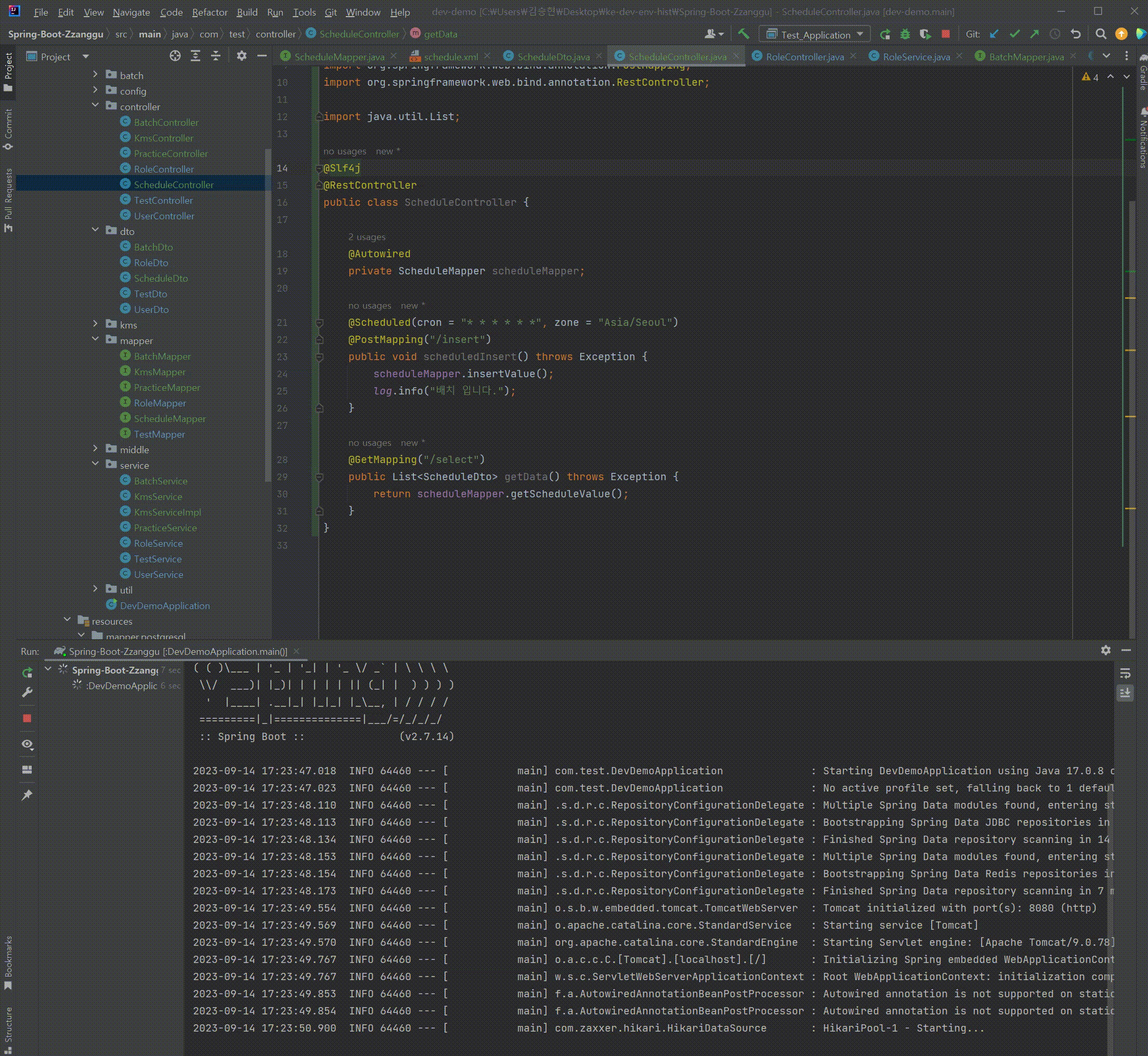
8. 결과
- 필요한 정보만 따로 모아놓은 API를 개발해서 보면 좋을거 같긴 하다.
예를들어 오늘의 날씨 라던지, 아니면 특정 주식의 가격이라던지 정보를 매일매일 아니면 주기적으로 업데이트를 받아서 개인 API로 활용하면 좋을거 같다.
반응형
'⭐ SpringBoot > 🕜 Spring Schedule (스프링 스케줄)' 카테고리의 다른 글
SpringBatch 테스트 코드 및 실행 (0) | 2023.09.12 |
---|